Note
Go to the end to download the full example code.
Keypoints#
This example shows how to create basic geometry using keypoints commands.
This section is focused on creating keypoints.
import numpy as np
from ansys.mapdl.core import launch_mapdl
# start MAPDL and enter the pre-processing routine
mapdl = launch_mapdl()
mapdl.clear()
mapdl.prep7()
print(mapdl)
Mapdl
-----
PyMAPDL Version: 0.69.3
Interface: grpc
Product: Ansys Mechanical Enterprise Academic Student
MAPDL Version: 24.2
Running on: localhost
(127.0.0.1)
APDL Command: K#
Create a single keypoint at [0, 0, 0]
. Note that the first
value is an empty string to allow MAPDL to pick the keypoint number.
1
Create keypoint at (10, 11, 12) while specifying the keypoint number.
2
APDL Command: KBETW#
Create keypoint between two keypoints
3
APDL Command: KCENTER#
Create keypoint at the center of a circular arc defined by three locations. Note that we first clear mapdl before generating this geometry
[1, 2, 3, 4]
Keypoint IDs#
Return an array of the keypoint IDs
Note that this matches the array [k0, k1, k2, k3]
(due to sorting)
array([1, 2, 3, 4], dtype=int32)
Keypoints geometry#
Get the VTK MultiBlock
containing keypoints. This VTK mesh can be
saved or plotted. For more information, visit
PyVista documentation.
keypoints = mapdl.geometry.keypoints
keypoints
Keypoint Coordinates#
Return an array of the keypoint locations
APDL Command: KDIST#
Calculate the distance between two keypoints. Note that you could
compute this yourself from kloc
[1.414213562, 1.0, -1.0, 0.0]
Keypoint Selection#
There are two approaches for selecting keypoints, the old “legacy” style and the new style. The old style is valuable for those who are comfortable with the existing MAPDL commands, and new style is useful for selecting keypoints in a pythonic manner.
This example generates a series of random keypoints and selects them
mapdl.clear()
mapdl.prep7()
# create 20 random keypoints
for _ in range(20):
mapdl.k("", *np.random.random(3))
# Print the keypoint numbers
print(mapdl.geometry.knum)
[ 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20]
Select every other keypoint with the old style command.
mapdl.ksel("S", "KP", "", 1, 20, 2)
print(mapdl.geometry.knum)
[ 1 3 5 7 9 11 13 15 17 19]
Select every other keypoint with the new style command.
Note that the item IDs are 1 based in MAPDL, while Python ranges are 0 based.
mapdl.geometry.keypoint_select(range(1, 21, 2))
print(mapdl.geometry.knum)
[ 1 3 5 7 9 11 13 15 17 19]
Select keypoints from a list
Note that you can return_selected
if you want to see what you
have selected. This is helpful when reselecting from existing
areas.
Note that you could use a numpy array here as well.
[ 1 5 10 20]
APDL Command: KPLOT#
Plot the keypoints while displaying the keypoint numbers
There are a variety of plotting options available for all the common plotting methods.
mapdl.kplot(
show_keypoint_numbering=True,
background="white",
show_bounds=True,
font_size=26,
)
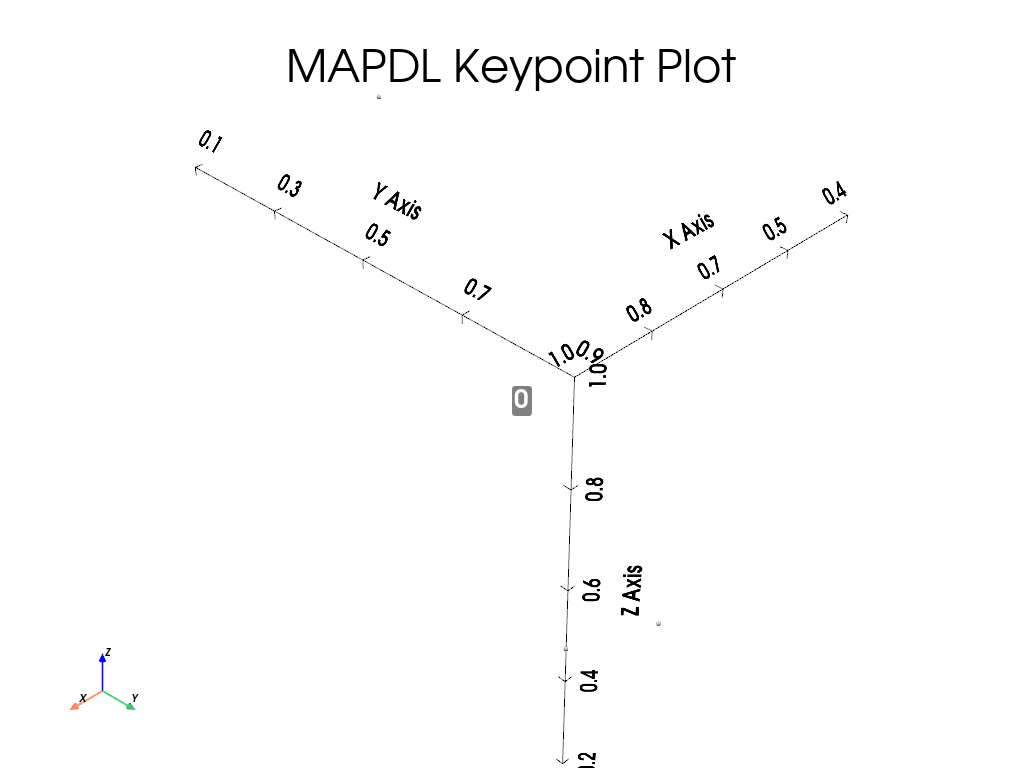
Stop mapdl#
mapdl.exit()
Total running time of the script: (0 minutes 1.146 seconds)