Mesh and geometry#
The Mapdl
class allows you to access
the mesh and geometry without writing to an intermediate file or
interpreting the text output from various MAPDL commands. For
example, to access the nodes and elements of a model, normally you
would list the nodes within MAPDL using the
Mapdl.nlist()
method.
However, this generates a string.
Array access requires either cumbersome MAPDL GET commands or that the
nodes be written to an archive file and then read in with other
software:
NLIST
LIST ALL SELECTED NODES. DSYS= 0
NODE X Y Z THXY THYZ THZX
1 0.0000 0.0000 0.0000 0.00 0.00 0.00
2 1.0000 0.0000 0.0000 0.00 0.00 0.00
3 0.2500 0.0000 0.0000 0.00 0.00 0.00
However, with the Mapdl.mesh
class,
you can interface with a current instance of the
Mapdl
class and access the current nodes coordinates
with this code:
>>> mapdl.mesh.nodes
[[0.0, 0.0, 0.0],
[1.0, 0.0, 0.0],
[0.25, 0.0, 0.0],
[0.75, 0.5, 3.5],
[0.75, 0.5, 4.0],
[0.75, 0.5, 4.5]]
Both the Mapdl.geometry
and
Mapdl.mesh
attributes support
additional, lower-level access to MAPDL data. You can use this code
to access them:
>>> mapdl.mesh
>>> mapdl.geometry
To view the current mesh status, you can use this code:
>>> mapdl.mesh
ANSYS Mesh
Number of Nodes: 7217
Number of Elements: 2080
Number of Element Types: 2
Number of Node Components: 0
Number of Element Components: 0
Geometry#
In PyMAPDL 0.66.0 and later, by default, all geometry entities are returned
as a pyvista.MultiBlock
object.
Example 1
>>> mapdl.geometry.areas
MultiBlock (0x147ca7640)
N Blocks 28
X Bounds -0.016, 0.016
Y Bounds -0.008, 0.018
Z Bounds -0.003, 0.015
Example 2:
>>> mapdl.geometry.keypoints
MultiBlock (0x147a78220)
N Blocks 26
X Bounds -0.016, 0.016
Y Bounds -0.008, 0.018
Z Bounds -0.003, 0.015
As you can see, you do not need to call the entities in the new API.
For more differences between the new Geometry API and the old one, see Migration to the new Geometry API.
The selection now is easier.
You can use indexing:
>>> volume0 = mapdl.geometry.volumes[0]
>>> volume0
UnstructuredGrid (0x149107340)
N Cells: 34
N Points: 36
X Bounds: 0.000e+00, 1.588e-02
Y Bounds: -7.620e-03, 1.778e-02
Z Bounds: -3.180e-03, 0.000e+00
N Arrays: 3
You can use the entity name:
>>> volume1 = mapdl.geometry.volumes["volume 1"]
>>> volume1
UnstructuredGrid (0x149107340)
N Cells: 34
N Points: 36
X Bounds: 0.000e+00, 1.588e-02
Y Bounds: -7.620e-03, 1.778e-02
Z Bounds: -3.180e-03, 0.000e+00
N Arrays: 3
You can plot different entities by calling the plot()
method:
>>> mapdl.geometry.areas.plot()
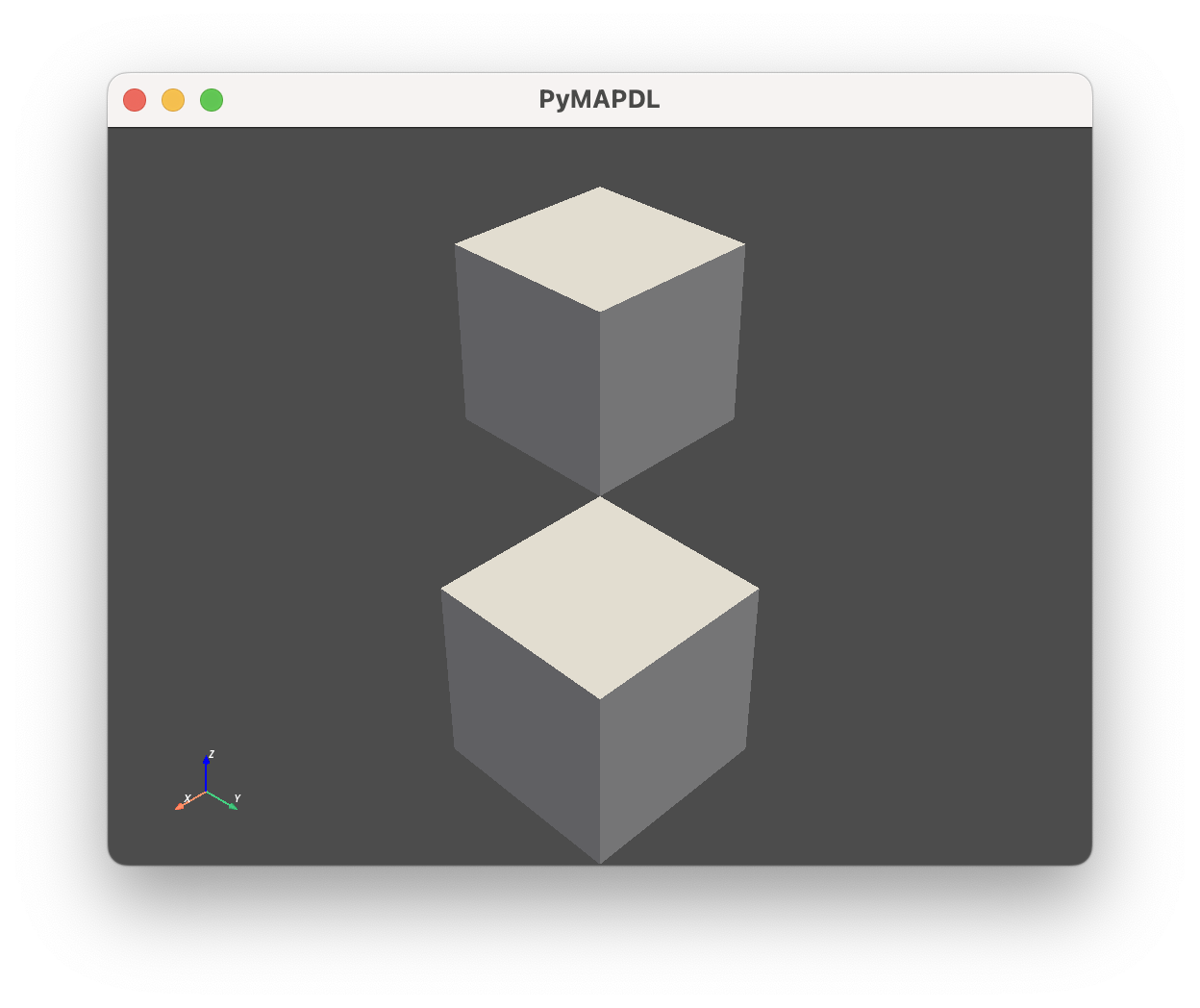
You can plot a single entity:
>>> mapdl.geometry.areas["area 1"].plot()
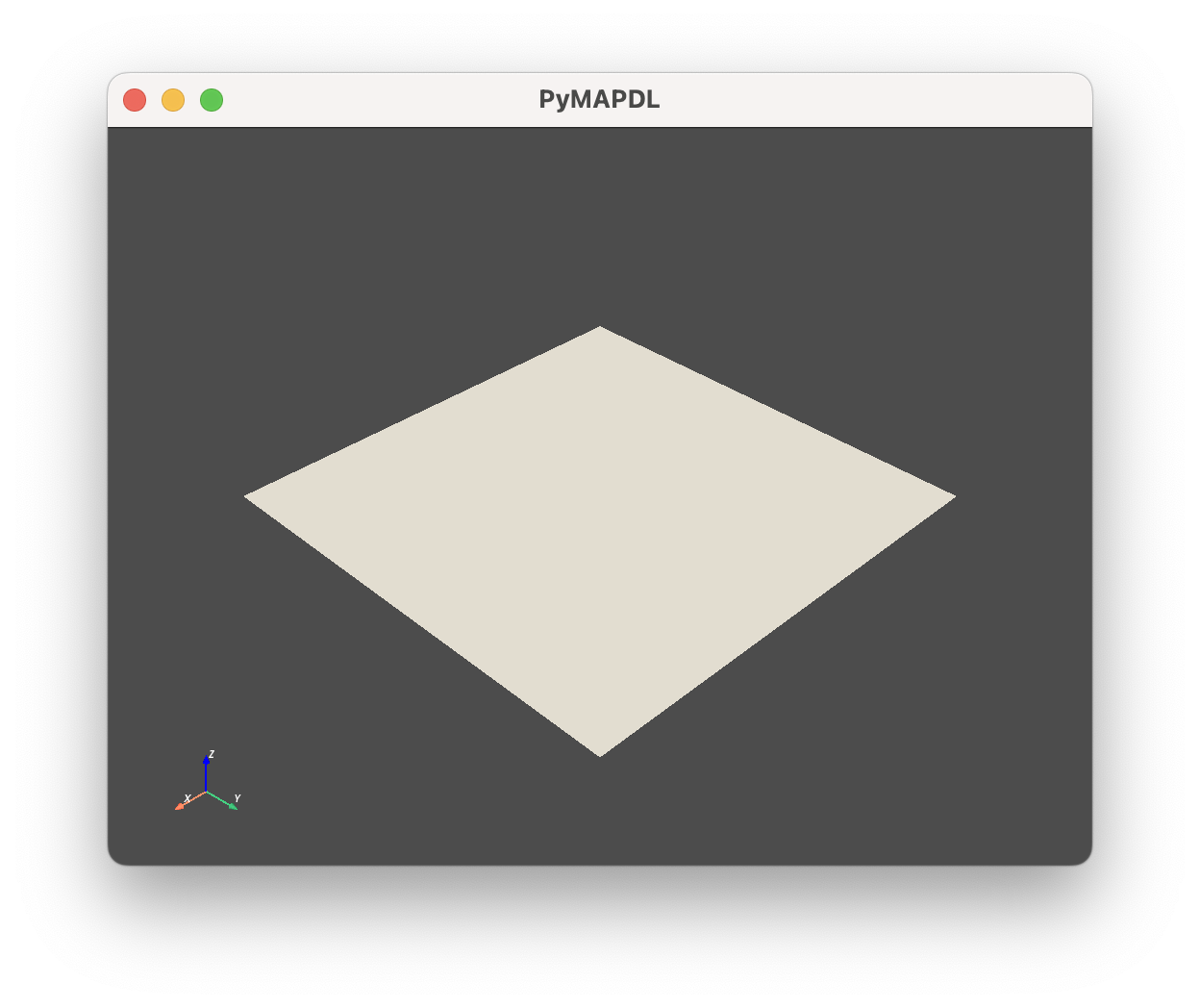
You can plot multiple entities using slices:
>>> mapdl.geometry.areas[2:12:2].plot()
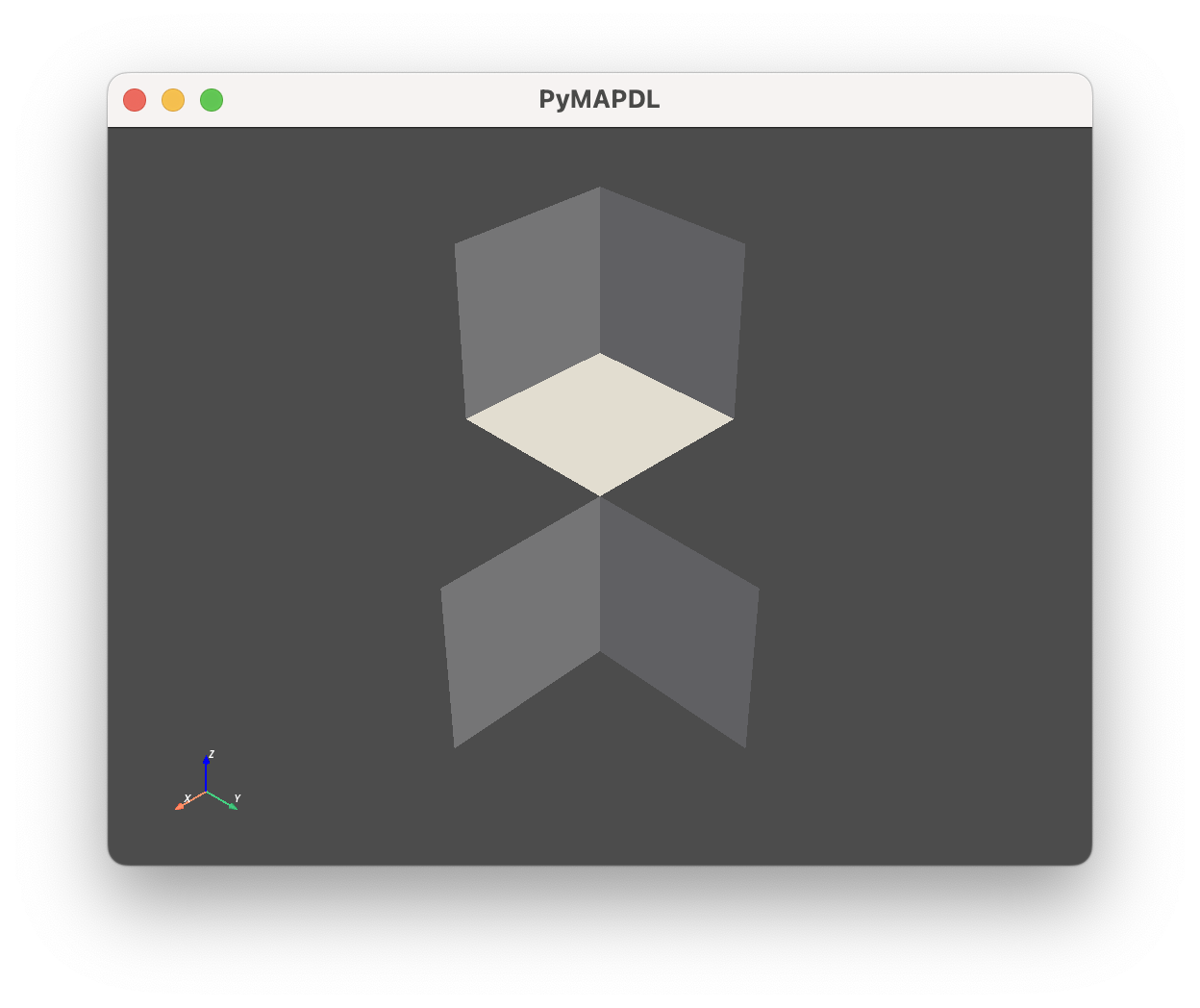
Furthermore, the following methods are provided to return the geometry entities as other Python objects:
Table 1. Get_ENTITY methods.
Default output |
|
|
|
|
---|---|---|---|---|
|
|
|||
Not Applicable |
Not Applicable |
|||
Not Applicable |
Not Applicable |
|||
Not Applicable |
Not Applicable |
Migration to the new Geometry API#
Several changes must be addressed in older scripts to update them from the old API to the new API.
One of the most important is that you no longer need to call the entities like you did in the old API.
Old API
# Old API
>>> mapdl.geometry.areas()
[UnstructuredGrid (0x7f14add95040)
N Cells: 12
N Points: 20
X Bounds: -2.000e+00, 2.000e+00
Y Bounds: 0.000e+00, 1.974e+00
Z Bounds: 0.000e+00, 0.000e+00
N Arrays: 4,
UnstructuredGrid (0x7f14add95ca0)
N Cells: 12
N Points: 20
X Bounds: -2.000e+00, 2.000e+00
Y Bounds: 0.000e+00, 1.974e+00
Z Bounds: 5.500e-01, 5.500e-01
N Arrays: 4,
...
New API
>>> mapdl.geometry.areas
MultiBlock (0x147ca7640)
N Blocks 28
X Bounds -0.016, 0.016
Y Bounds -0.008, 0.018
Z Bounds -0.003, 0.015
In addition, the type of entities returned by those methods are different. This table compares the objects returned by the old and new APIs:
Table 2. Comparison between objects returned by both APIs.
Function |
Old API (Function based - Must be called) |
New API (Property based - Doesn’t need to be called) |
---|---|---|
|
||
Not existent |
This table shows the equivalence between the old and new APIs:
Table 3. Equivalence between both API methods.
Old API |
New API equivalent |
---|---|
Not existent |
MAPDL geometry commands#
For additional MAPDL commands for creating geometries, see the Preprocessing commands.
API reference#
For a full description of the Mesh
and Geometry
classes,
see Mesh and Geometry.