Note
Go to the end to download the full example code.
Structural Analysis of a Lathe Cutter#
Basic walk through PyMAPDL capabilities.
Objective#
The objective of this example is to highlight some regularly used PyMAPDL features via a lathe cutter finite element model. Lathe cutters have multiple avenues of wear and failure, and the analyses supporting their design would most often be transient thermal-structural. However, for simplicity, this simulation example uses a non-uniform load.
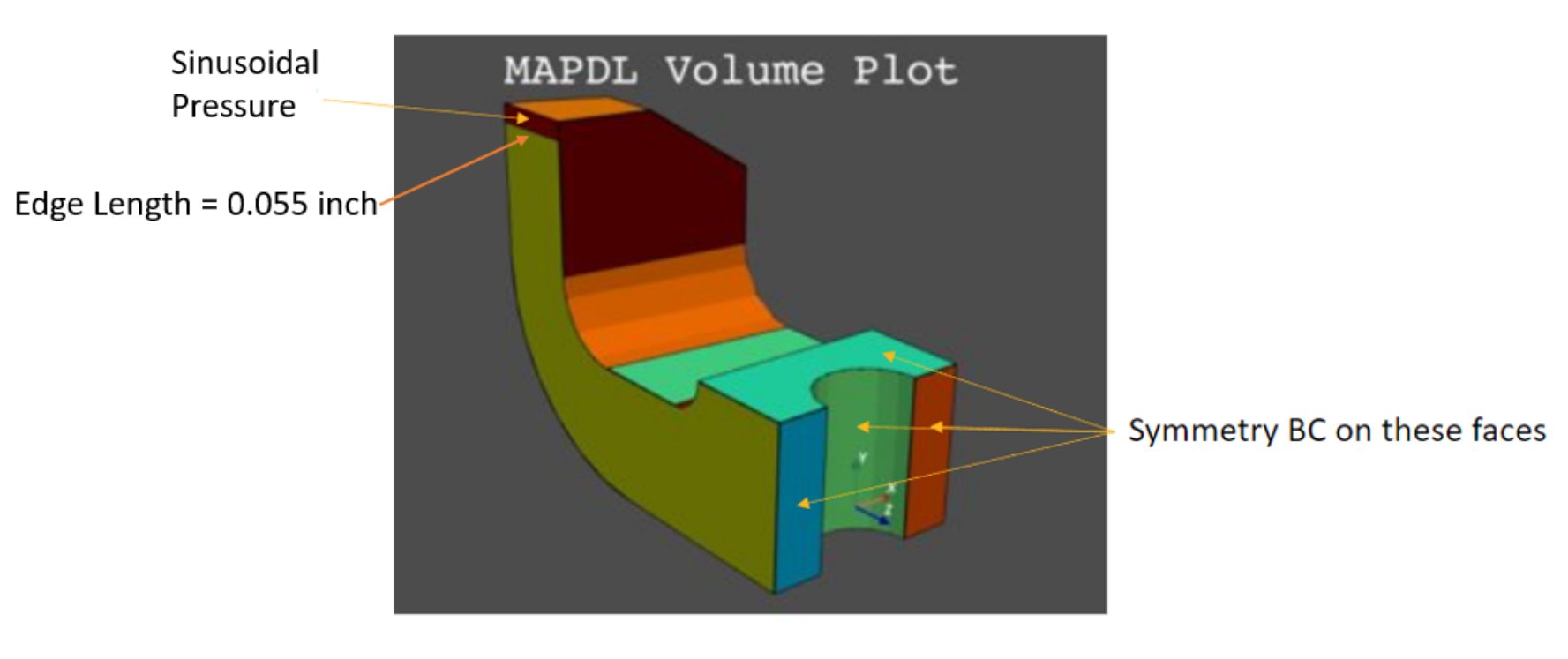
Figure 1: Lathe cutter geometry and load description.#
Contents#
Variables and launch Define necessary variables and launch MAPDL.
Geometry, mesh, and MAPDL parameters Import geometry and inspect for MAPDL parameters. Define linear elastic material model with Python variables. Mesh and apply symmetry boundary conditions.
Coordinate system and load Create a local coordinate system for the applied load and verify with a plot.
Pressure load Define the pressure load as a sine function of the length of the application area using numpy arrays. Import the pressure array into MAPDL as a table array. Verify the applied load and solve.
Plotting Show result plotting, plotting with selection, and working with the plot legend.
Postprocessing: List a result two ways: use PyMAPDL and the Pythonic version of APDL. Demonstrate extended methods and writing a list to a file.
Advanced plotting Use of
pyvista.UnstructuredGrid
for additional postprocessing.
Step 1: Variables and launch#
Define variables and launch MAPDL.
Often used MAPDL command line options are exposed as Pythonic parameter names in
ansys.mapdl.core.launcher.launch_mapdl()
. For example, -dir
has become run_location
.
You could use run_location
to specify the MAPDL run location. For example:
mapdl = launch_mapdl(run_location=path)
Otherwise, the MAPDL working directory is stored in mapdl.directory
. In this
directory, MAPDL will create some of the images we will show later.
Options without a Pythonic version can be accessed by the additional_switches
parameter.
Here -smp
is used only to keep the number of solver files to a minimum.
mapdl = launch_mapdl(additional_switches="-smp")
Step 2: Geometry, mesh, and MAPDL parameters#
Import geometry and inspect for MAPDL parameters.
Define material and mesh, and then create boundary conditions.
# First, reset the MAPDL database.
mapdl.clear()
Import the geometry file and list any MAPDL parameters.
lathe_cutter_geo = download_example_data("LatheCutter.anf", "geometry")
mapdl.input(lathe_cutter_geo)
mapdl.finish()
print(mapdl.parameters)
MAPDL Parameters
----------------
PRESS_LENGTH : 0.055
UNIT_SYSTEM : "bin"
Use pressure area per length in the load definition.
pressure_length = mapdl.parameters["PRESS_LENGTH"]
print(mapdl.parameters)
MAPDL Parameters
----------------
PRESS_LENGTH : 0.055
UNIT_SYSTEM : "bin"
Change the units and title.
mapdl.units("Bin")
mapdl.title("Lathe Cutter")
TITLE=
Lathe Cutter
Set material properties.
MATERIAL 1 NUXY = 0.2700000
The MAPDL element type SOLID285
is used for demonstration purposes.
Consider using an appropriate element type or mesh density for your actual
application.
mapdl.et(1, 285)
mapdl.smrtsize(4)
mapdl.aesize(14, 0.0025)
mapdl.vmesh(1)
mapdl.da(11, "symm")
mapdl.da(16, "symm")
mapdl.da(9, "symm")
mapdl.da(10, "symm")
CONSTRAINT AT AREA 10
LOAD LABEL = SYMM
Step 3: Coordinate system and load#
Create a local Coordinate System (CS) for the applied pressure as a function of local X.
Local CS ID is 11
mapdl.cskp(11, 0, 2, 1, 13)
mapdl.csys(1)
mapdl.view(1, -1, 1, 1)
mapdl.psymb("CS", 1)
mapdl.vplot(
color_areas=True,
show_lines=True,
cpos=[-1, 1, 1],
smooth_shading=True,
)
VTK plots do not show MAPDL plot symbols.
However, to use MAPDL plotting capabilities, you can set the keyword
option vtk
to False
.
mapdl.lplot(vtk=False)
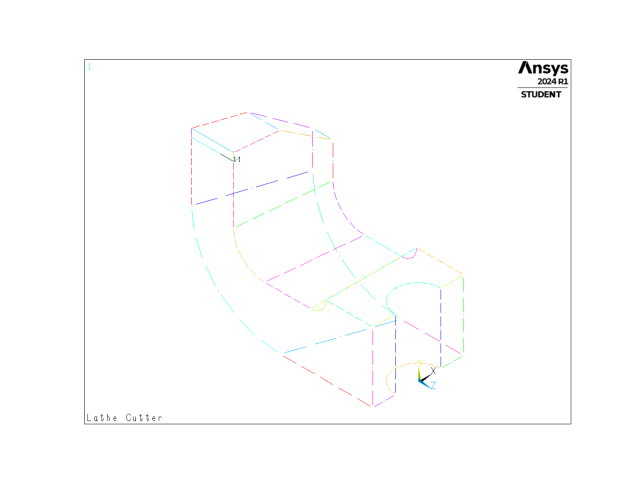
Step 4: Pressure load#
Create a pressure load, load it into MAPDL as a table array, verify the load, and solve.
length_x
and press
are vectors. To combine them into the correct
form needed to define the MAPDL table array, you can use
numpy.stack.
SELECT ALL ENTITIES OF TYPE= ALL AND BELOW
You can open the MAPDL GUI to check the model.
mapdl.open_gui()
Set up the solution.
mapdl.finish()
mapdl.slashsolu()
mapdl.nlgeom("On")
mapdl.psf("PRES", "NORM", 3, 0, 1)
mapdl.view(1, -1, 1, 1)
mapdl.eplot(vtk=False)
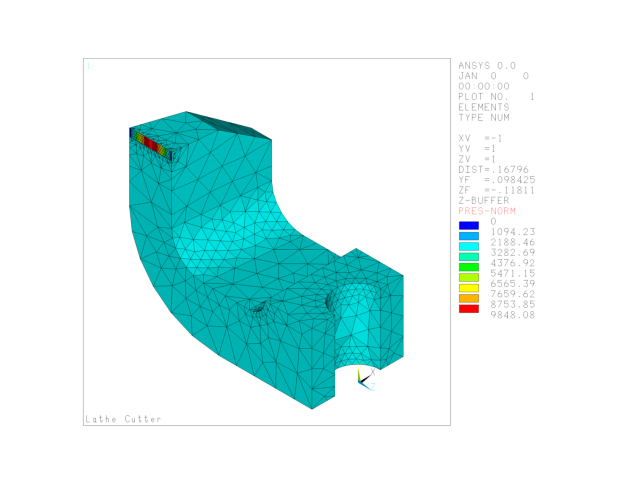
Solve the model.
mapdl.solve()
mapdl.finish()
if mapdl.solution.converged:
print("The solution has converged.")
The solution has converged.
Step 5: Plotting#
mapdl.post1()
mapdl.set("last")
mapdl.allsel()
mapdl.post_processing.plot_nodal_principal_stress("1", smooth_shading=False)
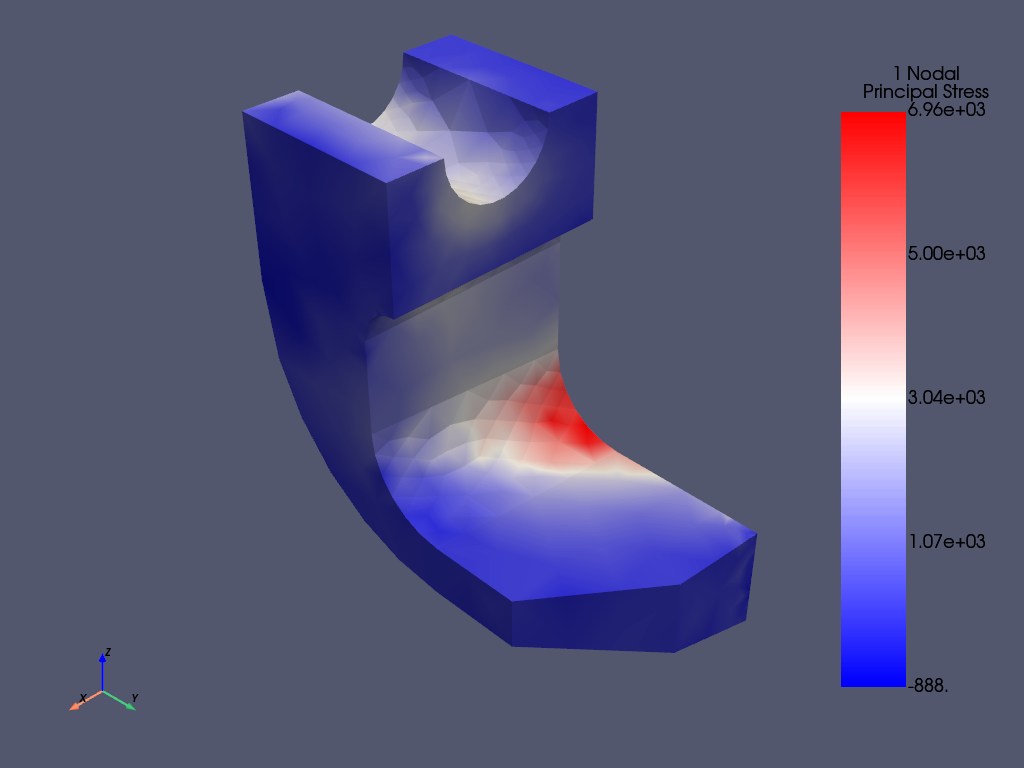
Plotting - Part of Model#
mapdl.csys(1)
mapdl.nsel("S", "LOC", "Z", -0.5, -0.141)
mapdl.esln()
mapdl.nsle()
mapdl.post_processing.plot_nodal_principal_stress(
"1", edge_color="white", show_edges=True
)
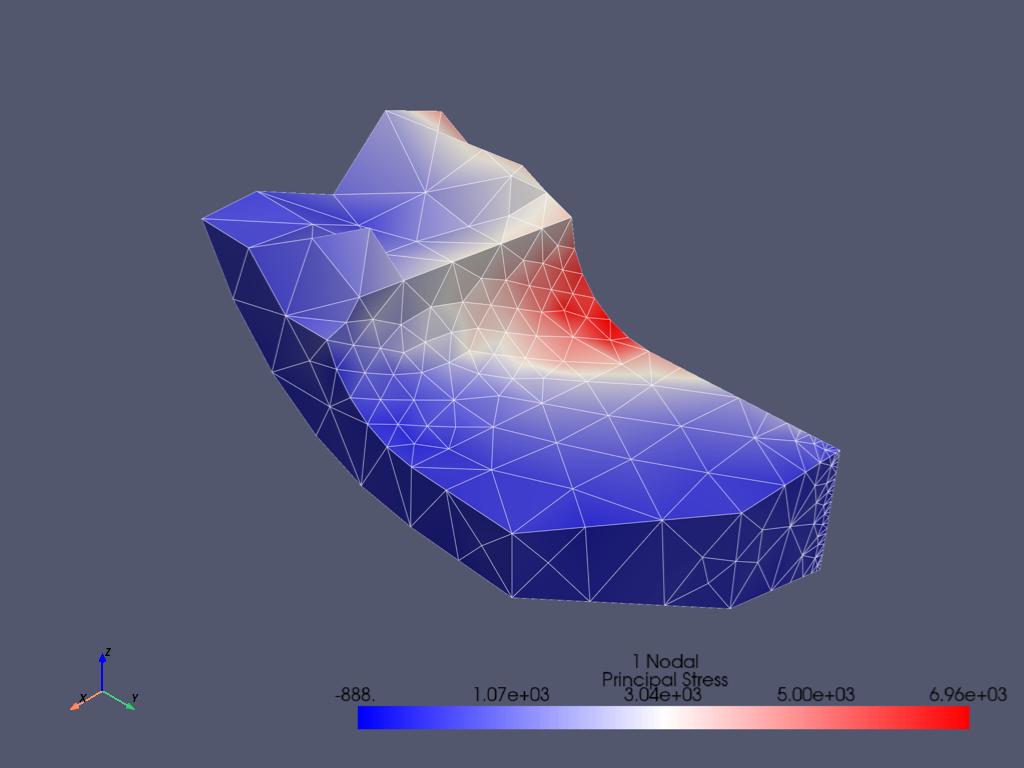
Plotting - Legend Options#
mapdl.allsel()
sbar_kwargs = {
"color": "black",
"title": "1st Principal Stress (psi)",
"vertical": False,
"n_labels": 6,
}
mapdl.post_processing.plot_nodal_principal_stress(
"1",
cpos="xy",
background="white",
edge_color="black",
show_edges=True,
scalar_bar_args=sbar_kwargs,
n_colors=9,
)
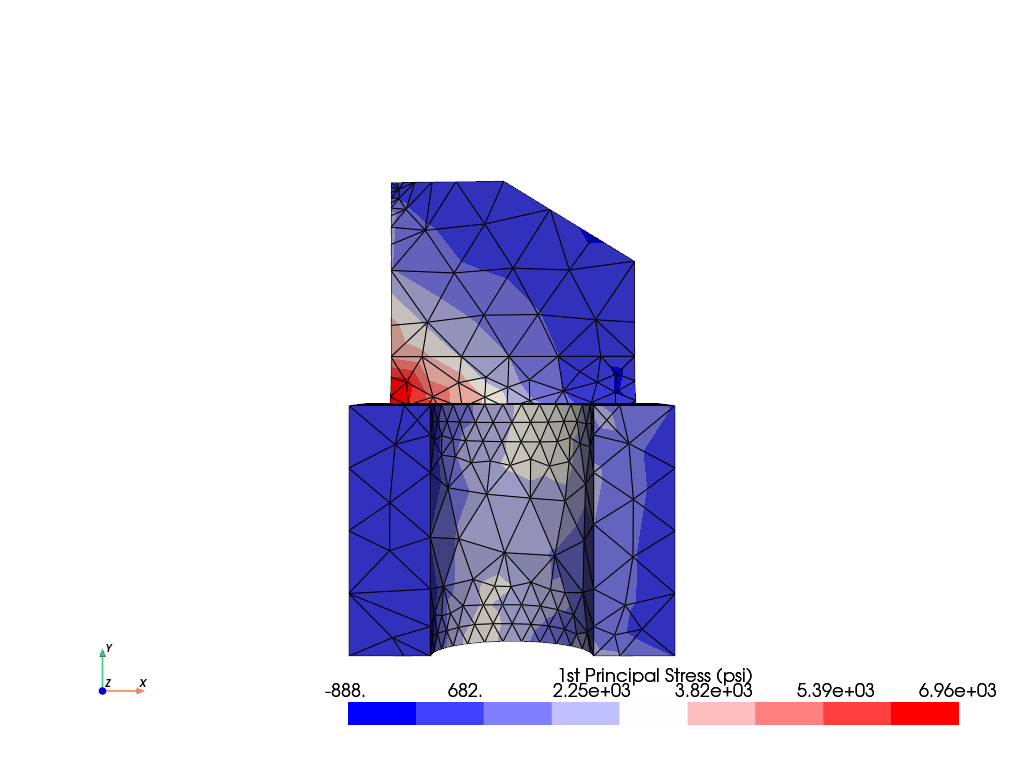
Let’s try out some scalar bar options from the PyVista documentation. For example, let’s set black text on a beige background.
The scalar bar keywords defined as a Python dictionary are an alternate method to using {key:value}’s. You can use the click-and drag method to reposition the scalar bar. Left-click it and hold down the left mouse button while moving the mouse.
sbar_kwargs = dict(
title_font_size=20,
label_font_size=16,
shadow=True,
n_labels=9,
italic=True,
bold=True,
fmt="%.1f",
font_family="arial",
title="1st Principal Stress (psi)",
color="black",
)
mapdl.post_processing.plot_nodal_principal_stress(
"1",
cpos="xy",
edge_color="black",
background="beige",
show_edges=True,
scalar_bar_args=sbar_kwargs,
n_colors=256,
cmap="jet",
)
# cmap names *_r usually reverses values. Try cmap='jet_r'
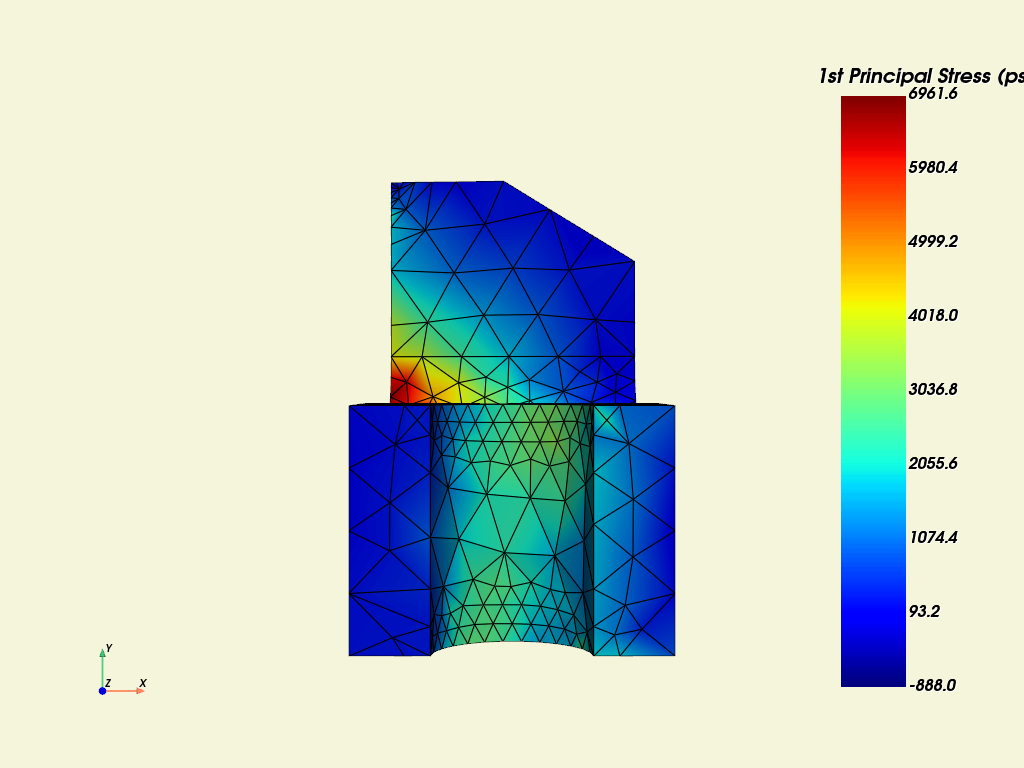
Step 6: Postprocessing#
Results List#
Get all principal nodal stresses.
mapdl.post_processing.nodal_principal_stress("1")
array([1095.57191833, 1162.29978117, 245.4551588 , ..., 1671.88717044,
2256.97869911, 1949.82365643])
Get the principal nodal stresses of the node subset.
mapdl.nsel("S", vmin=1200, vmax=1210)
mapdl.esln()
mapdl.nsle()
print("The node numbers are:")
print(mapdl.mesh.nnum) # get node numbers
print("The principal nodal stresses are:")
mapdl.post_processing.nodal_principal_stress("1")
The node numbers are:
[ 18 97 98 168 169 248 251 255 265 361 362 364 368 419
422 423 441 442 452 468 526 661 666 678 806 831 860 903
918 919 920 928 929 931 958 967 970 993 994 996 997 1007
1008 1039 1042 1050 1051 1055 1062 1063 1068 1069 1079 1089 1091 1094
1095 1096 1108 1110 1111 1112 1113 1115 1116 1117 1119 1120 1122 1126
1129 1155 1163 1164 1166 1177 1180 1181 1184 1185 1188 1190 1194 1195
1196 1197 1198 1200 1201 1202 1203 1204 1205 1206 1207 1208 1209 1210
1217 1224 1225 1226 1227 1229 1232 1238]
The principal nodal stresses are:
array([ 293.55119342, 360.98718875, 548.7375669 , 58.53449938,
862.13619361, 1710.3437169 , 1887.32668088, 1807.24198212,
1728.93198985, 684.04093398, 973.90565793, 1835.02798428,
996.83286654, -87.477287 , 556.60319322, 581.93533995,
481.31445511, 723.59293859, 1153.36222414, 886.50859761,
403.85334723, 439.8459606 , 495.32384548, 403.63059375,
316.10100914, 767.87856302, 851.38706331, 1190.28758079,
1485.68078124, 1103.20892574, 1543.48347154, 1288.91353874,
130.22051633, 738.60183259, 1897.42680197, 1481.8955267 ,
388.66516805, 1297.10202937, 1408.26632515, 838.64717812,
587.49559568, 1687.51244332, 1445.72818426, 1249.9561887 ,
1455.3714412 , 1366.61822021, 1391.90483489, 1625.1769307 ,
1144.05679862, 1327.46680635, 1027.89586754, 1112.87639164,
1554.05911789, 1029.33397703, 773.36444309, 1367.93897077,
1122.18758911, 1341.21534661, 2016.14764269, 1378.00544809,
1544.88823819, 1533.71938467, 1201.68162147, 901.51487977,
977.59388835, 1480.45133566, 1281.69835448, 1220.49842819,
1150.13518877, 732.76675627, 358.89782204, 1300.24561257,
1117.61991579, 1459.75358164, 1159.47137203, 797.90469382,
1378.37814687, 1375.62754117, 1148.71763083, 553.21194545,
1446.05670129, 1378.23911884, 1519.39456035, 1406.48652515,
1587.45177522, 1397.54957538, 1302.10961245, 1753.27639711,
1533.14150521, 1330.80797544, 1421.77663744, 1344.33692758,
349.82554218, 679.74835079, 1591.73190186, 1079.95284751,
650.07045872, 1186.27918715, 1732.23066805, 1247.57440958,
997.70961778, 1443.05183943, 1291.27365035, 1549.3411336 ,
1356.28591966, 1843.37098876])
Results as lists, arrays, and DataFrames#
Using mapdl.prnsol()
to check
print(mapdl.prnsol("S", "PRIN"))
PRINT S NODAL SOLUTION PER NODE
*****MAPDL VERIFICATION RUN ONLY*****
DO NOT USE RESULTS FOR PRODUCTION
***** POST1 NODAL STRESS LISTING *****
LOAD STEP= 1 SUBSTEP= 1
TIME= 1.0000 LOAD CASE= 0
NODE S1 S2 S3 SINT SEQV
18 293.55 -102.62 -2887.1 3180.7 3002.3
97 360.99 -213.63 -2333.8 2694.8 2458.3
98 548.74 -168.76 -1934.4 2483.2 2213.4
168 58.534 -256.76 -3523.5 3582.0 3435.3
169 862.14 -78.567 -2440.1 3302.2 2946.7
248 1710.3 297.57 -90.587 1800.9 1641.6
251 1887.3 697.04 9.7055 1877.6 1645.4
255 1807.2 506.74 -103.39 1910.6 1690.3
265 1728.9 698.37 -235.92 1964.8 1702.3
361 684.04 -265.84 -1685.9 2370.0 2065.9
362 973.91 -62.209 -1468.9 2442.8 2123.6
364 1835.0 201.41 -874.31 2709.3 2362.9
368 996.83 -22.522 -1429.2 2426.0 2109.9
419 -87.477 -521.45 -3963.3 3875.8 3678.1
422 556.60 -424.40 -3508.7 4065.3 3674.4
423 581.94 -28.195 -4049.7 4631.6 4358.7
441 481.31 -174.42 -3199.5 3680.9 3400.7
442 723.59 -94.017 -3282.2 4005.8 3666.0
452 1153.4 119.02 -2228.8 3382.2 3001.8
468 886.51 116.64 -1649.6 2536.1 2252.1
526 403.85 -183.16 -2258.5 2662.3 2422.8
661 439.85 -423.07 -2517.5 2957.4 2634.1
666 495.32 -552.58 -2092.2 2587.5 2254.3
678 403.63 -217.10 -2285.4 2689.1 2438.7
806 316.10 -552.31 -2831.2 3147.3 2815.4
831 767.88 -171.80 -2277.7 3045.6 2701.2
860 851.39 -183.94 -1419.4 2270.8 1969.1
903 1190.3 -110.73 -1056.8 2247.0 1954.1
918 1485.7 6.3415 -1856.8 3342.5 2901.1
919 1103.2 -271.42 -1858.5 2961.8 2567.2
920 1543.5 -117.29 -1895.2 3438.7 2978.6
928 1288.9 -63.544 -1765.8 3054.7 2651.3
929 130.22 -492.02 -3309.5 3439.7 3174.7
931 738.60 -710.70 -2727.8 3466.4 3015.4
958 1897.4 121.77 -1652.0 3549.5 3073.9
967 1481.9 -126.44 -1618.1 3100.0 2685.3
970 388.67 -342.36 -2432.5 2821.2 2535.9
993 1297.1 -69.232 -673.94 1971.0 1748.9
994 1408.3 109.68 -527.75 1936.0 1708.9
*****MAPDL VERIFICATION RUN ONLY*****
DO NOT USE RESULTS FOR PRODUCTION
***** POST1 NODAL STRESS LISTING *****
LOAD STEP= 1 SUBSTEP= 1
TIME= 1.0000 LOAD CASE= 0
NODE S1 S2 S3 SINT SEQV
996 838.65 -104.11 -2011.8 2850.5 2515.3
997 587.50 -168.66 -2216.8 2804.3 2513.0
1007 1687.5 590.80 -195.46 1883.0 1638.1
1008 1445.7 160.70 -399.18 1844.9 1638.4
1039 1250.0 -42.298 -829.33 2079.3 1818.3
1042 1455.4 56.014 -827.70 2283.1 1993.9
1050 1366.6 30.323 -558.14 1924.8 1708.3
1051 1391.9 123.37 -710.12 2102.0 1833.4
1055 1625.2 534.59 -104.88 1730.1 1515.2
1062 1144.1 -211.78 -1888.8 3032.9 2631.4
1063 1327.5 -110.33 -1929.0 3256.5 2826.6
1068 1027.9 -226.19 -1858.9 2886.8 2507.2
1069 1112.9 -309.26 -2054.9 3167.7 2748.1
1079 1554.1 7.6816 -952.58 2506.6 2190.5
1089 1029.3 28.635 -1997.0 3026.3 2670.5
1091 773.36 -180.67 -2035.9 2809.3 2474.3
1094 1367.9 137.66 -511.70 1879.6 1653.5
1095 1122.2 -160.47 -956.86 2079.1 1816.9
1096 1341.2 -59.158 -872.78 2214.0 1939.7
1108 2016.1 295.76 -1732.8 3749.0 3250.4
1110 1378.0 16.329 -1069.9 2447.9 2124.4
1111 1544.9 -6.0350 -1039.8 2584.7 2253.3
1112 1533.7 44.281 -838.13 2371.8 2076.4
1113 1201.7 -136.72 -1286.4 2488.1 2156.8
1115 901.51 -181.05 -1951.1 2852.7 2494.3
1116 977.59 -182.22 -1391.3 2368.9 2051.7
1117 1480.5 318.87 -396.04 1876.5 1640.4
1119 1281.7 -61.077 -1839.8 3121.5 2712.1
1120 1220.5 -7.9548 -1925.8 3146.3 2746.4
1122 1150.1 -84.100 -1847.6 2997.7 2609.6
1126 732.77 -578.00 -1996.9 2729.7 2364.6
1129 358.90 -623.35 -2611.7 2970.6 2621.3
1155 1300.2 -89.537 -1741.4 3041.7 2637.4
1163 1117.6 -338.37 -1811.8 2929.4 2537.0
1164 1459.8 -281.91 -1685.6 3145.4 2729.2
1166 1159.5 -241.30 -1793.1 2952.5 2558.1
1177 797.90 -187.81 -2210.6 3008.5 2656.5
1180 1378.4 34.377 -818.26 2196.6 1918.1
1181 1375.6 49.208 -732.58 2108.2 1846.0
*****MAPDL VERIFICATION RUN ONLY*****
DO NOT USE RESULTS FOR PRODUCTION
***** POST1 NODAL STRESS LISTING *****
LOAD STEP= 1 SUBSTEP= 1
TIME= 1.0000 LOAD CASE= 0
NODE S1 S2 S3 SINT SEQV
1184 1148.7 -274.84 -1874.1 3022.8 2619.3
1185 553.21 -487.94 -2230.7 2783.9 2436.3
1188 1446.1 -27.180 -955.21 2401.3 2097.4
1190 1378.2 -20.625 -756.85 2135.1 1878.5
1194 1519.4 123.54 -572.17 2091.6 1844.9
1195 1406.5 179.12 -404.69 1811.2 1601.2
1196 1587.5 173.43 -762.20 2349.6 2048.9
1197 1397.5 23.468 -741.92 2139.5 1877.7
1198 1302.1 -42.291 -728.07 2030.2 1788.8
1200 1753.3 536.06 -115.22 1868.5 1642.7
1201 1533.1 82.256 -882.15 2415.3 2105.8
1202 1330.8 -71.842 -925.05 2255.9 1972.9
1203 1421.8 51.803 -678.37 2100.1 1846.7
1204 1344.3 -186.38 -1741.5 3085.8 2672.4
1205 349.83 -269.66 -3028.7 3378.6 3115.4
1206 679.75 -129.38 -2256.8 2936.6 2627.2
1207 1591.7 -138.70 -1747.2 3339.0 2892.3
1208 1080.0 -80.069 -1271.9 2351.8 2036.8
1209 650.07 -374.78 -2257.6 2907.6 2554.4
1210 1186.3 -174.00 -1904.1 3090.3 2682.7
1217 1732.2 -148.05 -1663.8 3396.0 2946.7
1224 1247.6 -118.49 -982.93 2230.5 1947.9
1225 997.71 -160.41 -1218.8 2216.5 1920.2
1226 1443.1 -99.023 -987.68 2430.7 2130.3
1227 1291.3 -152.91 -1103.4 2394.7 2088.5
1229 1549.3 -227.74 -1794.8 3344.1 2898.0
1232 1356.3 -98.844 -941.59 2297.9 2013.4
1238 1843.4 -69.019 -1799.8 3643.2 3156.4
MINIMUM VALUES
NODE 0 0 0 0 0
VALUE -87.477 -710.70 -4049.7 1730.1 1515.2
MAXIMUM VALUES
NODE 0 0 0 0 0
VALUE 2016.1 698.37 9.7055 4631.6 4358.7
Use this command to obtain the data as a list.
mapdl_s_1_list = mapdl.prnsol("S", "PRIN").to_list()
print(mapdl_s_1_list)
[[18.0, 293.55, -102.62, -2887.1, 3180.7, 3002.3], [97.0, 360.99, -213.63, -2333.8, 2694.8, 2458.3], [98.0, 548.74, -168.76, -1934.4, 2483.2, 2213.4], [168.0, 58.534, -256.76, -3523.5, 3582.0, 3435.3], [169.0, 862.14, -78.567, -2440.1, 3302.2, 2946.7], [248.0, 1710.3, 297.57, -90.587, 1800.9, 1641.6], [251.0, 1887.3, 697.04, 9.7055, 1877.6, 1645.4], [255.0, 1807.2, 506.74, -103.39, 1910.6, 1690.3], [265.0, 1728.9, 698.37, -235.92, 1964.8, 1702.3], [361.0, 684.04, -265.84, -1685.9, 2370.0, 2065.9], [362.0, 973.91, -62.209, -1468.9, 2442.8, 2123.6], [364.0, 1835.0, 201.41, -874.31, 2709.3, 2362.9], [368.0, 996.83, -22.522, -1429.2, 2426.0, 2109.9], [419.0, -87.477, -521.45, -3963.3, 3875.8, 3678.1], [422.0, 556.6, -424.4, -3508.7, 4065.3, 3674.4], [423.0, 581.94, -28.195, -4049.7, 4631.6, 4358.7], [441.0, 481.31, -174.42, -3199.5, 3680.9, 3400.7], [442.0, 723.59, -94.017, -3282.2, 4005.8, 3666.0], [452.0, 1153.4, 119.02, -2228.8, 3382.2, 3001.8], [468.0, 886.51, 116.64, -1649.6, 2536.1, 2252.1], [526.0, 403.85, -183.16, -2258.5, 2662.3, 2422.8], [661.0, 439.85, -423.07, -2517.5, 2957.4, 2634.1], [666.0, 495.32, -552.58, -2092.2, 2587.5, 2254.3], [678.0, 403.63, -217.1, -2285.4, 2689.1, 2438.7], [806.0, 316.1, -552.31, -2831.2, 3147.3, 2815.4], [831.0, 767.88, -171.8, -2277.7, 3045.6, 2701.2], [860.0, 851.39, -183.94, -1419.4, 2270.8, 1969.1], [903.0, 1190.3, -110.73, -1056.8, 2247.0, 1954.1], [918.0, 1485.7, 6.3415, -1856.8, 3342.5, 2901.1], [919.0, 1103.2, -271.42, -1858.5, 2961.8, 2567.2], [920.0, 1543.5, -117.29, -1895.2, 3438.7, 2978.6], [928.0, 1288.9, -63.544, -1765.8, 3054.7, 2651.3], [929.0, 130.22, -492.02, -3309.5, 3439.7, 3174.7], [931.0, 738.6, -710.7, -2727.8, 3466.4, 3015.4], [958.0, 1897.4, 121.77, -1652.0, 3549.5, 3073.9], [967.0, 1481.9, -126.44, -1618.1, 3100.0, 2685.3], [970.0, 388.67, -342.36, -2432.5, 2821.2, 2535.9], [993.0, 1297.1, -69.232, -673.94, 1971.0, 1748.9], [994.0, 1408.3, 109.68, -527.75, 1936.0, 1708.9], [996.0, 838.65, -104.11, -2011.8, 2850.5, 2515.3], [997.0, 587.5, -168.66, -2216.8, 2804.3, 2513.0], [1007.0, 1687.5, 590.8, -195.46, 1883.0, 1638.1], [1008.0, 1445.7, 160.7, -399.18, 1844.9, 1638.4], [1039.0, 1250.0, -42.298, -829.33, 2079.3, 1818.3], [1042.0, 1455.4, 56.014, -827.7, 2283.1, 1993.9], [1050.0, 1366.6, 30.323, -558.14, 1924.8, 1708.3], [1051.0, 1391.9, 123.37, -710.12, 2102.0, 1833.4], [1055.0, 1625.2, 534.59, -104.88, 1730.1, 1515.2], [1062.0, 1144.1, -211.78, -1888.8, 3032.9, 2631.4], [1063.0, 1327.5, -110.33, -1929.0, 3256.5, 2826.6], [1068.0, 1027.9, -226.19, -1858.9, 2886.8, 2507.2], [1069.0, 1112.9, -309.26, -2054.9, 3167.7, 2748.1], [1079.0, 1554.1, 7.6816, -952.58, 2506.6, 2190.5], [1089.0, 1029.3, 28.635, -1997.0, 3026.3, 2670.5], [1091.0, 773.36, -180.67, -2035.9, 2809.3, 2474.3], [1094.0, 1367.9, 137.66, -511.7, 1879.6, 1653.5], [1095.0, 1122.2, -160.47, -956.86, 2079.1, 1816.9], [1096.0, 1341.2, -59.158, -872.78, 2214.0, 1939.7], [1108.0, 2016.1, 295.76, -1732.8, 3749.0, 3250.4], [1110.0, 1378.0, 16.329, -1069.9, 2447.9, 2124.4], [1111.0, 1544.9, -6.035, -1039.8, 2584.7, 2253.3], [1112.0, 1533.7, 44.281, -838.13, 2371.8, 2076.4], [1113.0, 1201.7, -136.72, -1286.4, 2488.1, 2156.8], [1115.0, 901.51, -181.05, -1951.1, 2852.7, 2494.3], [1116.0, 977.59, -182.22, -1391.3, 2368.9, 2051.7], [1117.0, 1480.5, 318.87, -396.04, 1876.5, 1640.4], [1119.0, 1281.7, -61.077, -1839.8, 3121.5, 2712.1], [1120.0, 1220.5, -7.9548, -1925.8, 3146.3, 2746.4], [1122.0, 1150.1, -84.1, -1847.6, 2997.7, 2609.6], [1126.0, 732.77, -578.0, -1996.9, 2729.7, 2364.6], [1129.0, 358.9, -623.35, -2611.7, 2970.6, 2621.3], [1155.0, 1300.2, -89.537, -1741.4, 3041.7, 2637.4], [1163.0, 1117.6, -338.37, -1811.8, 2929.4, 2537.0], [1164.0, 1459.8, -281.91, -1685.6, 3145.4, 2729.2], [1166.0, 1159.5, -241.3, -1793.1, 2952.5, 2558.1], [1177.0, 797.9, -187.81, -2210.6, 3008.5, 2656.5], [1180.0, 1378.4, 34.377, -818.26, 2196.6, 1918.1], [1181.0, 1375.6, 49.208, -732.58, 2108.2, 1846.0], [1184.0, 1148.7, -274.84, -1874.1, 3022.8, 2619.3], [1185.0, 553.21, -487.94, -2230.7, 2783.9, 2436.3], [1188.0, 1446.1, -27.18, -955.21, 2401.3, 2097.4], [1190.0, 1378.2, -20.625, -756.85, 2135.1, 1878.5], [1194.0, 1519.4, 123.54, -572.17, 2091.6, 1844.9], [1195.0, 1406.5, 179.12, -404.69, 1811.2, 1601.2], [1196.0, 1587.5, 173.43, -762.2, 2349.6, 2048.9], [1197.0, 1397.5, 23.468, -741.92, 2139.5, 1877.7], [1198.0, 1302.1, -42.291, -728.07, 2030.2, 1788.8], [1200.0, 1753.3, 536.06, -115.22, 1868.5, 1642.7], [1201.0, 1533.1, 82.256, -882.15, 2415.3, 2105.8], [1202.0, 1330.8, -71.842, -925.05, 2255.9, 1972.9], [1203.0, 1421.8, 51.803, -678.37, 2100.1, 1846.7], [1204.0, 1344.3, -186.38, -1741.5, 3085.8, 2672.4], [1205.0, 349.83, -269.66, -3028.7, 3378.6, 3115.4], [1206.0, 679.75, -129.38, -2256.8, 2936.6, 2627.2], [1207.0, 1591.7, -138.7, -1747.2, 3339.0, 2892.3], [1208.0, 1080.0, -80.069, -1271.9, 2351.8, 2036.8], [1209.0, 650.07, -374.78, -2257.6, 2907.6, 2554.4], [1210.0, 1186.3, -174.0, -1904.1, 3090.3, 2682.7], [1217.0, 1732.2, -148.05, -1663.8, 3396.0, 2946.7], [1224.0, 1247.6, -118.49, -982.93, 2230.5, 1947.9], [1225.0, 997.71, -160.41, -1218.8, 2216.5, 1920.2], [1226.0, 1443.1, -99.023, -987.68, 2430.7, 2130.3], [1227.0, 1291.3, -152.91, -1103.4, 2394.7, 2088.5], [1229.0, 1549.3, -227.74, -1794.8, 3344.1, 2898.0], [1232.0, 1356.3, -98.844, -941.59, 2297.9, 2013.4], [1238.0, 1843.4, -69.019, -1799.8, 3643.2, 3156.4]]
Use this command to obtain the data as an array:
mapdl_s_1_array = mapdl.prnsol("S", "PRIN").to_array()
print(mapdl_s_1_array)
[[ 18. 293.55 -102.62 -2887.1 3180.7 3002.3 ]
[ 97. 360.99 -213.63 -2333.8 2694.8 2458.3 ]
[ 98. 548.74 -168.76 -1934.4 2483.2 2213.4 ]
[ 168. 58.534 -256.76 -3523.5 3582. 3435.3 ]
[ 169. 862.14 -78.567 -2440.1 3302.2 2946.7 ]
[ 248. 1710.3 297.57 -90.587 1800.9 1641.6 ]
[ 251. 1887.3 697.04 9.7055 1877.6 1645.4 ]
[ 255. 1807.2 506.74 -103.39 1910.6 1690.3 ]
[ 265. 1728.9 698.37 -235.92 1964.8 1702.3 ]
[ 361. 684.04 -265.84 -1685.9 2370. 2065.9 ]
[ 362. 973.91 -62.209 -1468.9 2442.8 2123.6 ]
[ 364. 1835. 201.41 -874.31 2709.3 2362.9 ]
[ 368. 996.83 -22.522 -1429.2 2426. 2109.9 ]
[ 419. -87.477 -521.45 -3963.3 3875.8 3678.1 ]
[ 422. 556.6 -424.4 -3508.7 4065.3 3674.4 ]
[ 423. 581.94 -28.195 -4049.7 4631.6 4358.7 ]
[ 441. 481.31 -174.42 -3199.5 3680.9 3400.7 ]
[ 442. 723.59 -94.017 -3282.2 4005.8 3666. ]
[ 452. 1153.4 119.02 -2228.8 3382.2 3001.8 ]
[ 468. 886.51 116.64 -1649.6 2536.1 2252.1 ]
[ 526. 403.85 -183.16 -2258.5 2662.3 2422.8 ]
[ 661. 439.85 -423.07 -2517.5 2957.4 2634.1 ]
[ 666. 495.32 -552.58 -2092.2 2587.5 2254.3 ]
[ 678. 403.63 -217.1 -2285.4 2689.1 2438.7 ]
[ 806. 316.1 -552.31 -2831.2 3147.3 2815.4 ]
[ 831. 767.88 -171.8 -2277.7 3045.6 2701.2 ]
[ 860. 851.39 -183.94 -1419.4 2270.8 1969.1 ]
[ 903. 1190.3 -110.73 -1056.8 2247. 1954.1 ]
[ 918. 1485.7 6.3415 -1856.8 3342.5 2901.1 ]
[ 919. 1103.2 -271.42 -1858.5 2961.8 2567.2 ]
[ 920. 1543.5 -117.29 -1895.2 3438.7 2978.6 ]
[ 928. 1288.9 -63.544 -1765.8 3054.7 2651.3 ]
[ 929. 130.22 -492.02 -3309.5 3439.7 3174.7 ]
[ 931. 738.6 -710.7 -2727.8 3466.4 3015.4 ]
[ 958. 1897.4 121.77 -1652. 3549.5 3073.9 ]
[ 967. 1481.9 -126.44 -1618.1 3100. 2685.3 ]
[ 970. 388.67 -342.36 -2432.5 2821.2 2535.9 ]
[ 993. 1297.1 -69.232 -673.94 1971. 1748.9 ]
[ 994. 1408.3 109.68 -527.75 1936. 1708.9 ]
[ 996. 838.65 -104.11 -2011.8 2850.5 2515.3 ]
[ 997. 587.5 -168.66 -2216.8 2804.3 2513. ]
[ 1007. 1687.5 590.8 -195.46 1883. 1638.1 ]
[ 1008. 1445.7 160.7 -399.18 1844.9 1638.4 ]
[ 1039. 1250. -42.298 -829.33 2079.3 1818.3 ]
[ 1042. 1455.4 56.014 -827.7 2283.1 1993.9 ]
[ 1050. 1366.6 30.323 -558.14 1924.8 1708.3 ]
[ 1051. 1391.9 123.37 -710.12 2102. 1833.4 ]
[ 1055. 1625.2 534.59 -104.88 1730.1 1515.2 ]
[ 1062. 1144.1 -211.78 -1888.8 3032.9 2631.4 ]
[ 1063. 1327.5 -110.33 -1929. 3256.5 2826.6 ]
[ 1068. 1027.9 -226.19 -1858.9 2886.8 2507.2 ]
[ 1069. 1112.9 -309.26 -2054.9 3167.7 2748.1 ]
[ 1079. 1554.1 7.6816 -952.58 2506.6 2190.5 ]
[ 1089. 1029.3 28.635 -1997. 3026.3 2670.5 ]
[ 1091. 773.36 -180.67 -2035.9 2809.3 2474.3 ]
[ 1094. 1367.9 137.66 -511.7 1879.6 1653.5 ]
[ 1095. 1122.2 -160.47 -956.86 2079.1 1816.9 ]
[ 1096. 1341.2 -59.158 -872.78 2214. 1939.7 ]
[ 1108. 2016.1 295.76 -1732.8 3749. 3250.4 ]
[ 1110. 1378. 16.329 -1069.9 2447.9 2124.4 ]
[ 1111. 1544.9 -6.035 -1039.8 2584.7 2253.3 ]
[ 1112. 1533.7 44.281 -838.13 2371.8 2076.4 ]
[ 1113. 1201.7 -136.72 -1286.4 2488.1 2156.8 ]
[ 1115. 901.51 -181.05 -1951.1 2852.7 2494.3 ]
[ 1116. 977.59 -182.22 -1391.3 2368.9 2051.7 ]
[ 1117. 1480.5 318.87 -396.04 1876.5 1640.4 ]
[ 1119. 1281.7 -61.077 -1839.8 3121.5 2712.1 ]
[ 1120. 1220.5 -7.9548 -1925.8 3146.3 2746.4 ]
[ 1122. 1150.1 -84.1 -1847.6 2997.7 2609.6 ]
[ 1126. 732.77 -578. -1996.9 2729.7 2364.6 ]
[ 1129. 358.9 -623.35 -2611.7 2970.6 2621.3 ]
[ 1155. 1300.2 -89.537 -1741.4 3041.7 2637.4 ]
[ 1163. 1117.6 -338.37 -1811.8 2929.4 2537. ]
[ 1164. 1459.8 -281.91 -1685.6 3145.4 2729.2 ]
[ 1166. 1159.5 -241.3 -1793.1 2952.5 2558.1 ]
[ 1177. 797.9 -187.81 -2210.6 3008.5 2656.5 ]
[ 1180. 1378.4 34.377 -818.26 2196.6 1918.1 ]
[ 1181. 1375.6 49.208 -732.58 2108.2 1846. ]
[ 1184. 1148.7 -274.84 -1874.1 3022.8 2619.3 ]
[ 1185. 553.21 -487.94 -2230.7 2783.9 2436.3 ]
[ 1188. 1446.1 -27.18 -955.21 2401.3 2097.4 ]
[ 1190. 1378.2 -20.625 -756.85 2135.1 1878.5 ]
[ 1194. 1519.4 123.54 -572.17 2091.6 1844.9 ]
[ 1195. 1406.5 179.12 -404.69 1811.2 1601.2 ]
[ 1196. 1587.5 173.43 -762.2 2349.6 2048.9 ]
[ 1197. 1397.5 23.468 -741.92 2139.5 1877.7 ]
[ 1198. 1302.1 -42.291 -728.07 2030.2 1788.8 ]
[ 1200. 1753.3 536.06 -115.22 1868.5 1642.7 ]
[ 1201. 1533.1 82.256 -882.15 2415.3 2105.8 ]
[ 1202. 1330.8 -71.842 -925.05 2255.9 1972.9 ]
[ 1203. 1421.8 51.803 -678.37 2100.1 1846.7 ]
[ 1204. 1344.3 -186.38 -1741.5 3085.8 2672.4 ]
[ 1205. 349.83 -269.66 -3028.7 3378.6 3115.4 ]
[ 1206. 679.75 -129.38 -2256.8 2936.6 2627.2 ]
[ 1207. 1591.7 -138.7 -1747.2 3339. 2892.3 ]
[ 1208. 1080. -80.069 -1271.9 2351.8 2036.8 ]
[ 1209. 650.07 -374.78 -2257.6 2907.6 2554.4 ]
[ 1210. 1186.3 -174. -1904.1 3090.3 2682.7 ]
[ 1217. 1732.2 -148.05 -1663.8 3396. 2946.7 ]
[ 1224. 1247.6 -118.49 -982.93 2230.5 1947.9 ]
[ 1225. 997.71 -160.41 -1218.8 2216.5 1920.2 ]
[ 1226. 1443.1 -99.023 -987.68 2430.7 2130.3 ]
[ 1227. 1291.3 -152.91 -1103.4 2394.7 2088.5 ]
[ 1229. 1549.3 -227.74 -1794.8 3344.1 2898. ]
[ 1232. 1356.3 -98.844 -941.59 2297.9 2013.4 ]
[ 1238. 1843.4 -69.019 -1799.8 3643.2 3156.4 ]]
or as a DataFrame:
mapdl_s_1_df = mapdl.prnsol("S", "PRIN").to_dataframe()
mapdl_s_1_df.head()
Use this command to obtain the data as a DataFrame, which is a. Pandas data type. Because the Pandas module is imported, you can use its functions. For example, you can write principal stresses to a file.
# mapdl_s_1_df.to_csv(path + '\prin-stresses.csv')
# mapdl_s_1_df.to_json(path + '\prin-stresses.json')
mapdl_s_1_df.to_html(path + "\prin-stresses.html")
Step 7: Advanced plotting#
mapdl.allsel()
principal_1 = mapdl.post_processing.nodal_principal_stress("1")
Load this result into the VTK grid.
grid = mapdl.mesh.grid
grid["p1"] = principal_1
sbar_kwargs = {
"color": "black",
"title": "1st Principal Stress (psi)",
"vertical": False,
"n_labels": 6,
}
Generate a single horizontal slice along the XY plane.
Note
PyVista’s eye_dome_lighting
method is used here to enhance the plots of the slices.
For more information, see`Eye Dome Lighting <pyvista_eye_dome_lighting>`_.
single_slice = grid.slice(normal=[0, 0, 1], origin=[0, 0, 0])
single_slice.plot(
scalars="p1",
background="white",
lighting=False,
eye_dome_lighting=True,
show_edges=False,
cmap="jet",
n_colors=9,
scalar_bar_args=sbar_kwargs,
)
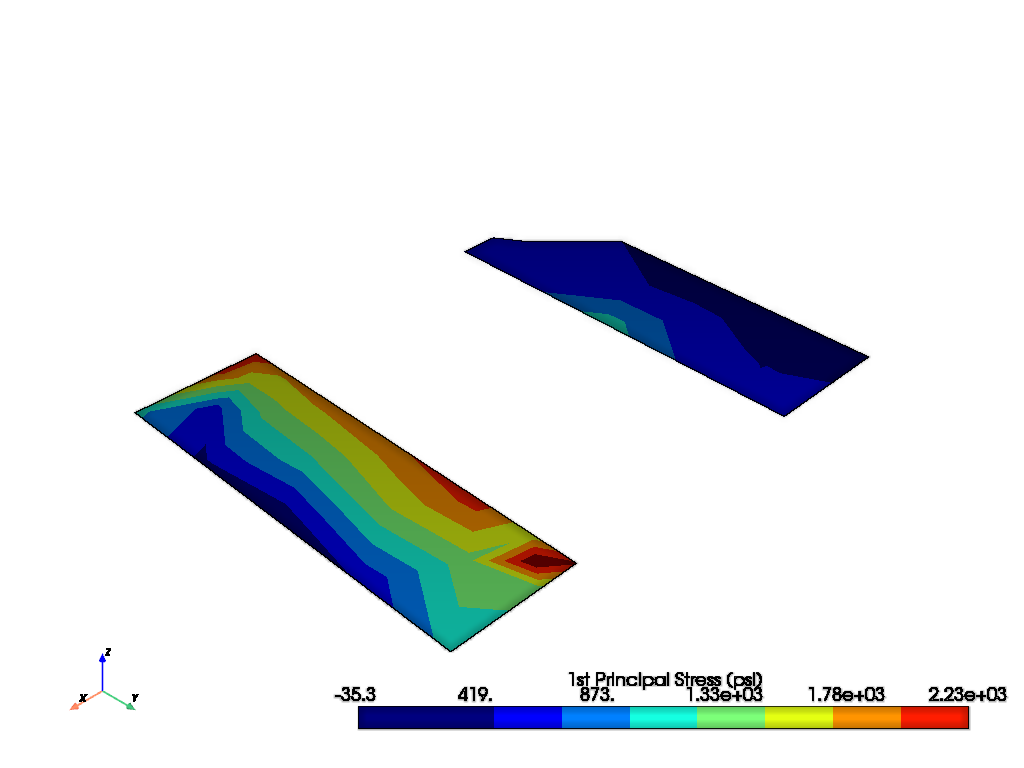
Generate a plot with three slice planes.
slices = grid.slice_orthogonal()
slices.plot(
scalars="p1",
background="white",
lighting=False,
eye_dome_lighting=True,
show_edges=False,
cmap="jet",
n_colors=9,
scalar_bar_args=sbar_kwargs,
)
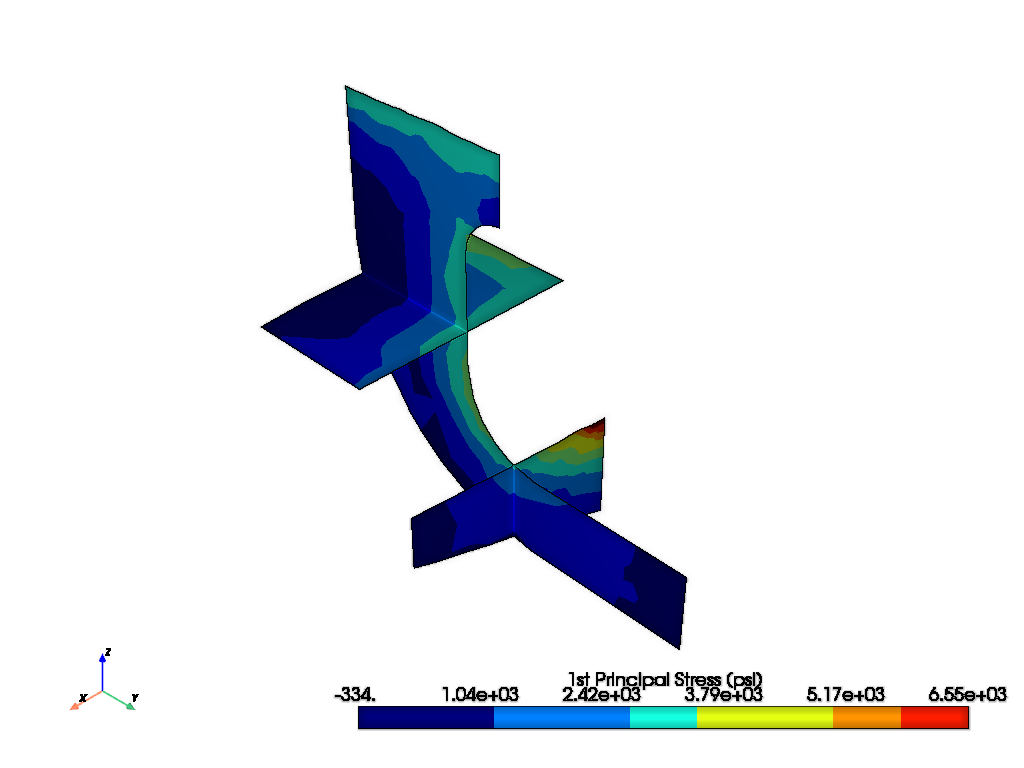
Generate a grid with multiple slices in the same plane.
slices = grid.slice_along_axis(12, "x")
slices.plot(
scalars="p1",
background="white",
show_edges=False,
lighting=False,
eye_dome_lighting=True,
cmap="jet",
n_colors=9,
scalar_bar_args=sbar_kwargs,
)
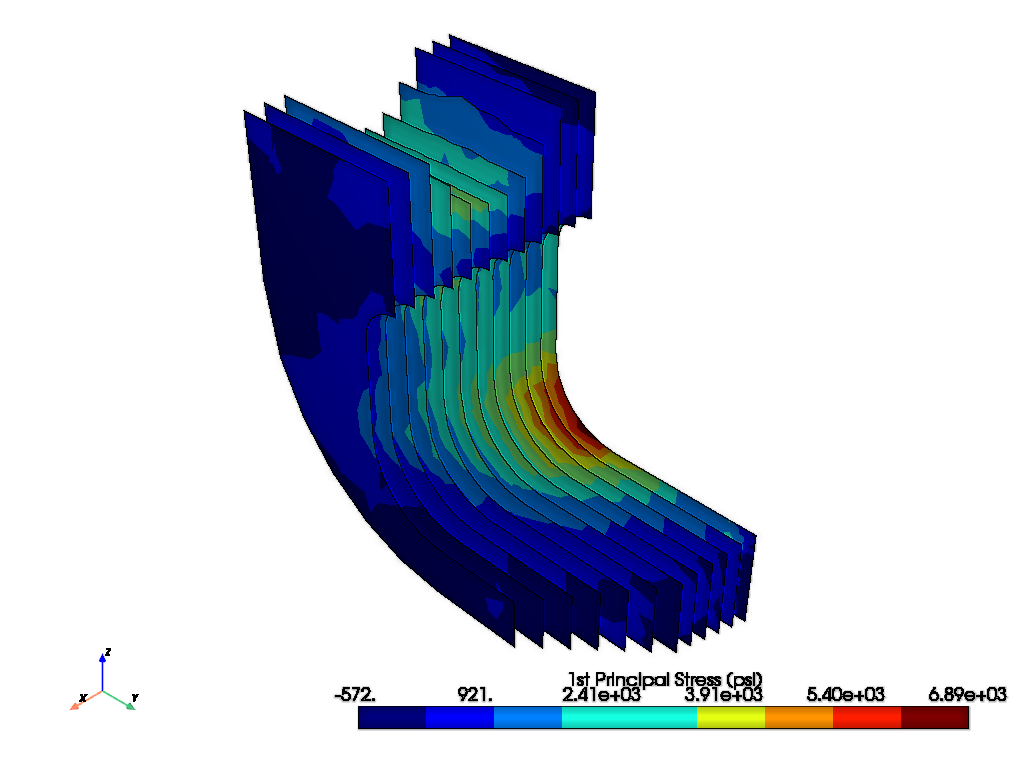
Finally, exit MAPDL.
mapdl.exit()
Total running time of the script: (0 minutes 46.800 seconds)